Send emails from Stripe Webhooks
Stripe is a business's best friend. It's a powerful platform that allows you to accept payment and manage your business. With Stripe webhooks, you can automate vast parts of your business. In this post, we'll show you how to use Stripe webhooks and Plunk to automatically send emails to your customers.
What is Plunk?
Plunk is the all-in-one email platform. It allows you to send transactional emails, marketing emails, and broadcasts from a single platform. Plunk contacts are shared across all of your emails. This means that you get a single view of your contacts through one single platform.
What are webhooks?
A webhook is a way for an application to communicate with another application. It's often used to notify other applications of important events. For example, Stripe uses webhooks to notify your application when a new subscription is made or a payment fails.
We can use these webhooks to automatically send emails to your customers. For example, we can send an email to new customers when they sign up or an email to customers when they cancel their subscription.
Setting up a webhook in Stripe
Setting up an endpoint
Webhooks are configured in the Stripe dashboard. To set up a webhook, go to the webhooks page and click on the Add endpoint button.
Stripe will send webhooks to the URL you specify. This means that you will either have to host your own API or use a third-party service like Zapier to process the webhooks. If you're using a custom API, then you can select your preferred language from the dropdown and Stripe will generate the code needed to process the webhooks.
Choosing the events
Stripe can send triggers for all sorts of events. You can choose which events you want to receive. For this tutorial we'll only be using the customer.subscription.created event. This event is triggered when a new subscription is created.
Select the event and click on the Add endpoint button. Your webhook is now set up and Stripe is ready to send events to your endpoint!

Setting up Plunk
To send emails with Plunk, you'll need to create a Plunk project. You can do this by going to the Plunk dashboard.
To send the emails, we will be using the marketing actions, these are automatic emails that are sent when Plunk receives an event. When first creating a project, you will be asked to complete an onboarding that will guide you through the process of setting up your first marketing action.
Sending an event to Plunk
Marketing automations start from events. You can send events to Plunk using the Plunk API. To do this, you'll need to copy your API key in the Plunk dashboard. Once you have your API key, you can use it to send an event like this.
We will be using a simplified version of the Node.js code that Stripe generates. You can find the full example in the webhook creation wizard.
const stripe = require('stripe')('sk_test_...');
const express = require('express');
const app = express();
const endpointSecret = "<WEBHOOK_SECRET>";
app.post('/webhook', express.raw({type: 'application/json'}), async (request, response) => {
const sig = request.headers['stripe-signature'];
const event = stripe.webhooks.constructEvent(request.body, sig, endpointSecret);
switch (event.type) {
case 'customer.subscription.created':
// Get the subscription object
const customerSubscriptionCreated = event.data.object;
// Get the customer email
const customer = await stripe.customers.retrieve(customerSubscriptionCreated.customer);
// Send an event to Plunk
await fetch('https://api.useplunk.com/v1/track', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer <API_KEY>',
},
body: JSON.stringify({
event: 'customer.subscription.created',
email: customer.email,
})
});
break;
}
response.send();
});
The code above will send an event to Plunk when a new subscription is created. Now that we have the event in Plunk we can use it to set up our marketing action.
Creating a template
The next step of the onboarding will take you through the process of creating a template. Templates are blueprints for your emails. You can add dynamic content to templates and easily edit them to create different emails.
You can try out Plunk's email editor without creating an account. You can find the editor on the markdown to html page.

Creating an action
With the event and template ready, we can create our marketing action. The action will be triggered when the event is received and will automatically send the email to the customer. Next to the event and template you can also optionally link more events or set up a delay.

That's it!
Now that we have everything set up, we can test it out. To test the webhook, we can use Stripe's CLI. You can find more information about the CLI and how to install it on the Stripe documentation.
Use the CLI to forward webhook events to your local server and create a new subscription. If everything went well, you should receive an email from Plunk with the template you created.
It's as easy as that. You can now use Plunk to send emails to your customers on any event. You can also use the webhook you created to keep your own database in sync with Stripe!
Resources
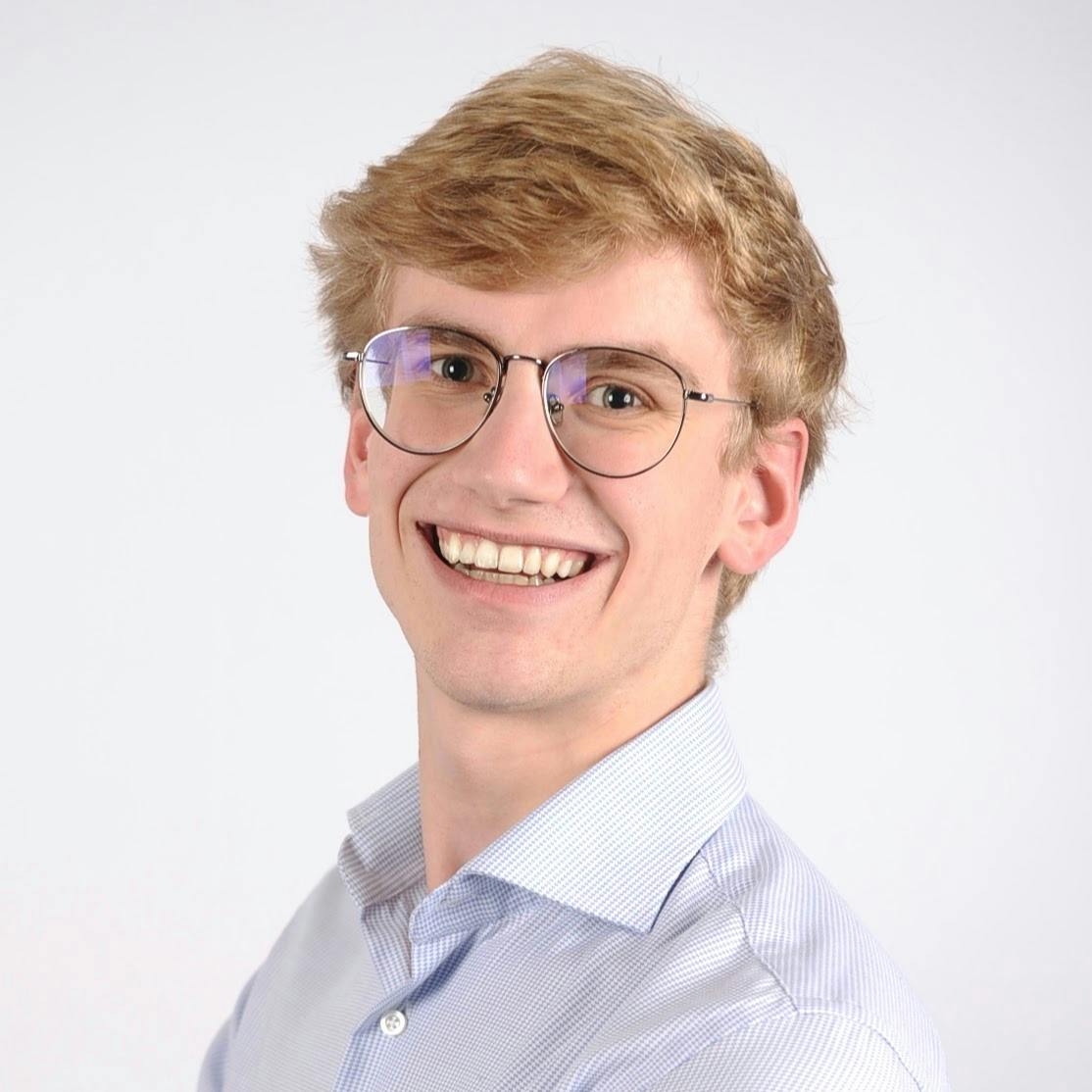