React Email with Plunk
React Email is the new kid on the block when it comes to creating and styling emails. Their intuitive set of React components makes it easy to create beautiful emails that look good on any device.
In this post, we'll show you how to use React Email together with the Plunk Transactional Email API to send beautiful emails to your users.
Installing packages
To start using React Email with Plunk, you'll need to install the following packages using your package manager of choice.
yarn add @plunk/node @react-email/render @react-email/html @react-email/button
Creating a template
Using React Email is easy. You can create a template by creating a new file and adding a new component to it. Components behave just like in React, they can have state and props.
Inside the component, you can leverage the installed React Email components to create your template. In our example we have installed the @react-email/html and @react-email/button packages. We can use these to create a simple template.
Some of the other components you can use are:
- @react-email/container
- @react-email/section
- @react-email/link
- @react-email/heading
import * as React from 'react';
import { Html } from '@react-email/html';
import { Button } from '@react-email/button';
export default function Email(props) {
const { url } = props;
return (
<Html lang="en">
<Button href={url}>Click me</Button>
</Html>
);
}
Converting and sending
Once your template is ready, all that is left to do is to convert it to HTML and send it using the Plunk Transactional Email API. Use the @react-email/render package to convert your template to HTML.
import { render } from '@react-email/render';
import { Email } from './Email';
import Plunk from '@plunk/node';
const plunk = new Plunk("YOUR_API_KEY");
const body = render(<Email url="https://example.com" />);
await plunk.emails.send({
to: "hello@useplunk.com",
subject: "Hello from Plunk",
body
});
Want to learn more?
If you want to learn more about Plunk, check out our documentation. More information about React Email, including a full list of components and templates, can be found on their website.
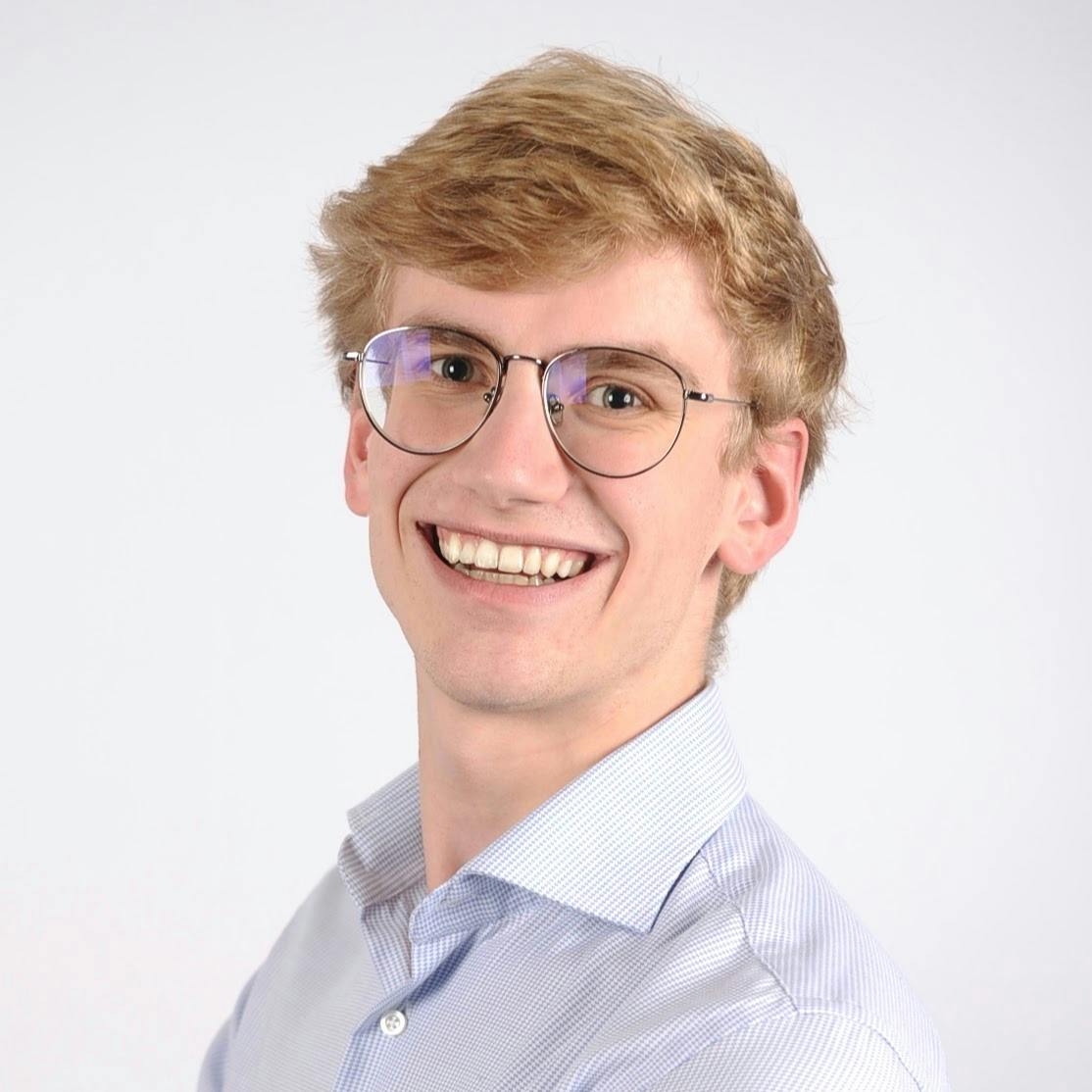